Azure AD business-to-business (B2B) collaboration capabilities enable any organization using Azure AD to work safely and securely with users from any other organization, small or large. Those organizations can be with Azure AD or without, or even with an IT organization or without. Organizations using Azure AD can provide access to documents, resources, and applications to their partners, while maintaining complete control over their own corporate data. Developers can use the Azure AD business-to-business APIs to write applications that bring two organizations together in more securely. Also, it's pretty easy for end users to navigate.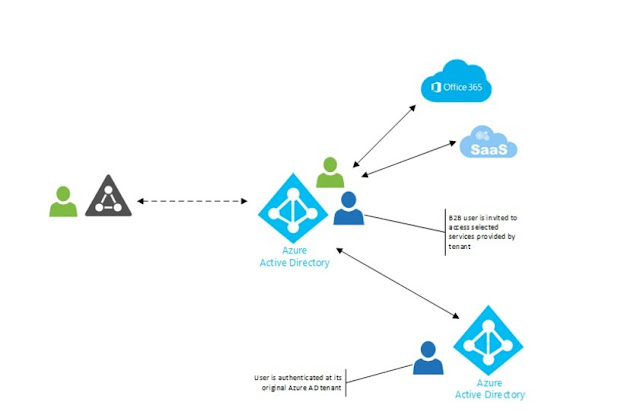
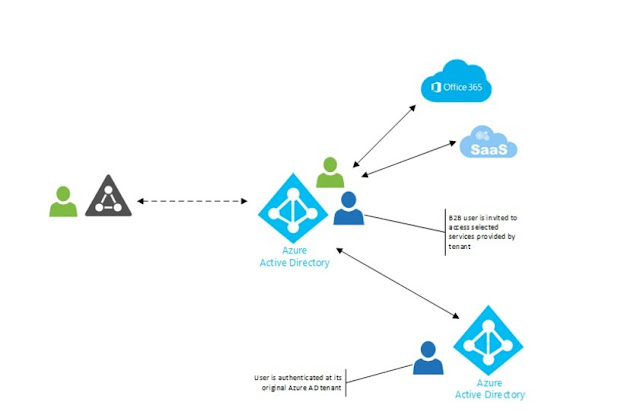
Cross-organization collaboration is hot and at the same time not so easy to roll. When you collaborate with an external party there are some things to be aligned:
- Is our security policy matching yours?
- Do we have to create an account for your users?
- If we give accounts to your users, who will disable it if needed?
- And who will take care about those pesky password resets?
Azure AD B2B aims to address this problem. When you
invite a user to your application, this user will get access using its Azure AD
account. No need to create an account for them. No need for a new password.
They sign-on to your app with their credentials. Hint: As stated earlier, Azure
is on its own controlled by Azure AD. If you want some external consultant to
gain access to your Azure instance, don’t use Microsoft Account for that.
Invite them with Azure B2B if they have their account in this service. On the
other hand, you are still in control of your application. You decide if it
requires multi-factor authentication. You choose who has the access. Azure AD
B2B provides API around it so you can build your on boarding process and send
invitations to apps. Or you can use the default one in the service. An
organization is using applications based on Azure AD and wants to collaborate
in these applications with some other businesses. Azure AD B2B allows working
together by granting access to these applications to users from another Azure
AD tenant.
The key benefits of Azure AD B2B collaboration to your organization
Provide access to any corporate app or data, while applying sophisticated, Azure AD-powered authorization policies
Enable your collaborators to bring their own identity B2B collaborators can sign in with an identity of their choice. If the user doesn’t have a Microsoft account or an Azure AD account – one is created for them seamlessly at the time for offer redemption.
Delegate to application and group owners Application and group owners can add B2B users directly to any application that you care about, whether it is a Microsoft application or not. Admin can delegate permission to add B2B users to non-admin. Non-admin can use the Azure AD Application Access Panel to add B2B collaboration users to applications or groups.
- Work with any user from any partner
- Partners use their own credentials
- No requirement for partners to use Azure AD
- No external directories or complex set-up required
- Simple and secure collaboration
Provide access to any corporate app or data, while applying sophisticated, Azure AD-powered authorization policies
- Easy for users
- Enterprise-grade security for apps and data
- No management overhead
- No external account or password management
- No sync or manual account lifecycle management
- No external administrative overhead
Enable your collaborators to bring their own identity B2B collaborators can sign in with an identity of their choice. If the user doesn’t have a Microsoft account or an Azure AD account – one is created for them seamlessly at the time for offer redemption.
Delegate to application and group owners Application and group owners can add B2B users directly to any application that you care about, whether it is a Microsoft application or not. Admin can delegate permission to add B2B users to non-admin. Non-admin can use the Azure AD Application Access Panel to add B2B collaboration users to applications or groups.
Procedure for invite your Guest User:
Required Permissions In Azure Portal :
In azure portal add permission for to invite users for azure applications.
Step-1:
First select Azure active directory in azure portal.
Step-2:
Then select App Registration then select your application.
Step-3:
Register the custom API
All requests to Microsoft Graph must be authorized. (The documentation discusses the authorization in depth.) In addition, the registered application must request permissions relevant to the operations they perform. The Create Invitation method requires Directory.ReadWrite.All or User.ReadWrite.All, both of which will require administrator consent. In addition, we must decide if the application will use Delegated permissions or Application permissions. Since the capability we are providing requires administrative privilege, and not every user has administrative privilege, application permissions are configured.
Our API does not require the typical user consent flow, nor does it require the permission to log in users. The application registration in Azure AD requests only the application permission Directory.ReadWrite.All,
We can perform the admin consent in the Azure portal, since we do not have a user login flow.
Application Permissions
Delegated Permissions:
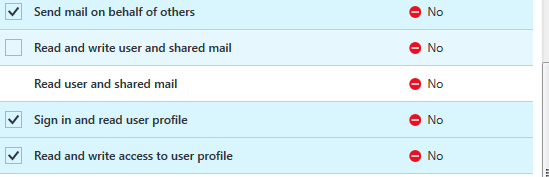
In ASP.Net Page:
Coding:
using System;
using System.Linq;
using System.Net.Http;
using System.Net.Http.Headers;
using Microsoft.IdentityModel.Clients.ActiveDirectory;
using Newtonsoft.Json;
namespace Graph_Api_User
{
public partial class WebForm1 : System.Web.UI.Page
{
string GraphResource = "https://graph.microsoft.com";
string InviteEndPoint = "https://graph.microsoft.com/v1.0/invitations";
string EstsLoginEndpoint = "https://login.microsoftonline.com";
string TenantID = "your-tenant-Id";
string TestAppClientId = "App_Id";
string TestAppClientSecret = "Secret
Key";
string InvitedUserEmailAddress = "abc@gmail.com";
string InvitedUserDisplayName = "abc";
protected void Page_Load(object sender, EventArgs e)
{
Invitation invitation = CreateInvitation();
SendInvitation(invitation);
}
private Invitation CreateInvitation()
{
// Set
the invitation object.
Invitation invitation = new Invitation();
invitation.InvitedUserDisplayName =
InvitedUserDisplayName;
invitation.InvitedUserEmailAddress
= InvitedUserEmailAddress;
invitation.InviteRedirectUrl = "https://myapps.microsoft.com";
invitation.SendInvitationMessage = true;
return invitation;
}
/// <summary>
/// Send the guest user invite request.
/// </summary>
/// <param
name="invitation">Invitation object.</param>
private void SendInvitation(Invitation invitation)
{
string accessToken = GetAccessToken();
HttpClient httpClient = GetHttpClient(accessToken);
// Make
the invite call.
HttpContent content = new StringContent(JsonConvert.SerializeObject(invitation));
content.Headers.Add("ContentType", "application/json");
var postResponse = httpClient.PostAsync(InviteEndPoint,
content).Result;
string serverResponse =
postResponse.Content.ReadAsStringAsync().Result;
Response.Write(serverResponse);
}
/// <summary>
/// Get the HTTP client.
/// </summary>
/// <param
name="accessToken">Access token</param>
/// <returns>Returns the Http Client.</returns>
private HttpClient GetHttpClient(string accessToken)
{
// setup
http client.
HttpClient httpClient = new HttpClient();
httpClient.Timeout = TimeSpan.FromSeconds(300);
httpClient.DefaultRequestHeaders.Authorization = new AuthenticationHeaderValue("Bearer", accessToken);
httpClient.DefaultRequestHeaders.Add("client-request-id", Guid.NewGuid().ToString());
Response.Write("CorrelationID for the request:"+httpClient.DefaultRequestHeaders.GetValues("client-request-id").Single());
return httpClient;
}
private string GetAccessToken()
{
string accessToken = null;
// Get
the access token for our application to talk to microsoft graph.
try
{
AuthenticationContext testAuthContext =
new AuthenticationContext(string.Format("{0}/{1}",
EstsLoginEndpoint, TenantID));
AuthenticationResult testAuthResult = testAuthContext.AcquireTokenAsync(
GraphResource,
new ClientCredential(TestAppClientId,
TestAppClientSecret)).Result;
accessToken =
testAuthResult.AccessToken;
}
catch (AdalException ex)
{
Response.Write("An exception was thrown while fetching the
token:"+ ex);
// throw;
}
return accessToken;
}
/// <summary>
/// Invitation class.
/// </summary>
public class Invitation
{
/// <summary>
/// Gets or sets display name.
/// </summary>
public string InvitedUserDisplayName { get; set; }
/// <summary>
/// Gets or sets display name.
/// </summary>
public string InvitedUserEmailAddress { get; set; }
/// <summary>
/// Gets or sets a value indicating whether Invitation
Manager should send the email to InvitedUser.
/// </summary>
public bool SendInvitationMessage { get; set; }
/// <summary>
/// Gets or sets invitation redirect URL
/// </summary>
public string InviteRedirectUrl { get; set; }
}
}
}
Limitations:
Multi-factor authentication (MFA) not supported on external users.- Invites are possible only via CSV; individual invites and API access are not supported.
- Only Azure AD Global Administrators can upload .csv files.
- Invitations to consumer email addresses (such as hotmail.com, Gmail.com, or comcast.net) are currently not supported.
- External user access to on-premises applications not tested.
- External users are not automatically cleaned up when the actual user is deleted from their directory.
- Invitations to DLs are not supported.
- Maximum of 2,000 records can be uploaded via CSV.
User Side Response:
If that user already a user in active directory, then it will go to the login screen.
After login, the user directly redirects to the page url.
0 Comments